前回はロードとイネーブル付きの蝶テキトーなカウンタしかださなかったので、少しマトモなモノにしてみる。"すとりーむ"な信号処理をメインにメシを喰っているので、その辺りから。比較的簡単なブツで、しかもVHDLっぽいモノっつー訳で移動平均フィルタにしますか。
はい、まずは数学的基礎。離散時間表現では、
\overline{x[k + L]} = 1/N \sum_{n = 0}^{N} x[k - n]
ですな。移動平均長がNで実装上のレイテンシーがLね。そんだけ。
じゃあ、実装です。とりあえず、こうしようぜ。
\overline{x[k + L]} = (x[k] + x[k-1] + ... + x[k - N]) / N
完璧ですね、と思ったアナタには漏れ無くパンチをプレゼント。 :DDD
次の様に漸化式にするのが、王道です。
\delta S[k + L_1] = x[k] - x[k - L]
S[k + L_2] = S[k] + \delta S[k]
\overline{x[k + L_3]} = S[k] / N
さらに実装を容易にする為、Nを2の冪乗に限定する事で除算を算術右シフトにします。
\delta S[k + L_1] = x[k] - x[k - N]
S[k + L_2] = S[k] + \delta S[k]
\overline{x[k + L_3]} = SAR(S[k], log_{2}(N))
つまり、移動平均長Nだけ離れた入力の差分を積算してテキトーに上位ビットを切り出すだけと言う簡単な回路。
あまりに簡単過ぎるので、もう少し内容を盛り込む。
まずは、instantiateする場合にcomponent declarationを書くのがダルいのでpackageを作って、use clauseを使う。つぎに、architecture bodyで使うsignalをrecordを使ってムニゃる。あと、synchronous/asynchronous resetに対応する。
以下、ソース。
1 --はい、簡単ですね。 :)
2 -- Moving Average Filter
3 --
4
5 library ieee;
6 use ieee.std_logic_1164.all;
7
8 package MAF_PKG is
9
10 component MAF is
11 generic (
12 IS_SYNC : boolean := true;
13 DW : integer range 2 to integer'high := 12;
14 log2N : integer range 1 to integer'high := 3
15 );
16 port (
17 iCLK : in std_logic;
18 iCLR : in std_logic;
19 iD : in std_logic_vector(DW-1 downto 0);
20 oQ : out std_logic_vector(DW-1 downto 0)
21 );
22 end component MAF;
23
24 end package MAF_PKG;
25
26 package body MAF_PKG is
27
28 end package body MAF_PKG;
29
30 library ieee;
31 use ieee.std_logic_1164.all;
32 use ieee.std_logic_arith.all;
33
34 entity MAF is
35 generic (
36 IS_SYNC : boolean := true;
37 DW : integer range 2 to integer'high := 12;
38 log2N : integer range 1 to integer'high := 3
39 );
40 port (
41 iCLK : in std_logic;
42 iCLR : in std_logic;
43 iD : in std_logic_vector(DW-1 downto 0);
44 oQ : out std_logic_vector(DW-1 downto 0)
45 );
46 begin
47 end entity MAF;
48
49 architecture TP of MAF is
50
51 constant cN : integer range 2 to integer'high := 2**log2N+1;
52
53 type tD is array (0 to cN-1) of std_logic_vector(DW-1 downto 0);
54 type t is record
55 D : tD;
56 dS : std_logic_vector( DW downto 0);
57 dSE : std_logic_vector(log2N+DW-1 downto 0);
58 S : std_logic_vector(log2N+DW-1 downto 0);
59 Q : std_logic_vector( DW-1 downto 0);
60 end record t;
61
62 constant c : t := (
63 D => (others => (others => '0')),
64 dS => (others => '0'),
65 dSE => (others => '0'),
66 S => (others => '0'),
67 Q => (others => '0')
68 );
69
70 signal g : t;
71 signal r : t := c;
72
73 begin
74
75 P_COMB : process (iD, r)
76 variable v : t;
77 begin
78 -- NOTE: Shift inputted data (D).
79 F_SHIFT_D : for index in 0 to cN-1 loop
80 if (index = 0) then
81 v.D(index) := iD;
82 else
83 v.D(index) := r.D(index-1);
84 end if;
85 end loop F_SHIFT_D;
86
87 -- NOTE: Compute delta sum (dS).
88 v.dS :=
89 signed(r.D( 0)(DW-1) & r.D( 0)) -
90 signed(r.D(cN-1)(DW-1) & r.D(cN-1));
91
92 -- NOTE: Sign expansion for delta sum (dSE).
93 v.dSE(log2N+DW-1 downto DW) := (log2N -1 downto 0 => r.dS(DW));
94 v.dSE( DW-1 downto 0) := r.dS( DW-1 downto 0);
95
96 -- NOTE: Accumulate sum (S).
97 v.S := signed(r.S) + signed(r.dSE);
98
99 -- NOTE: Divide sum by length for MA (Q).
100 v.Q := r.S(log2N+DW-1 downto log2N);
101
102 g <= v;
103 oQ <= r.Q;
104 end process P_COMB;
105
106 G_SYNC : if (IS_SYNC = true) generate
107 begin
108 P_SEQ : process (iCLK)
109 begin
110 if (iCLK'event and iCLK = '1') then
111 if (iCLR = '1') then
112 r <= c;
113 else
114 r <= g;
115 end if;
116 end if;
117 end process P_SEQ;
118 end generate G_SYNC;
119
120 G_ASYNC : if (IS_SYNC = false) generate
121 begin
122 P_SEQ : process (iCLR, iCLK)
123 begin
124 if (iCLR = '1') then
125 r <= c;
126 elsif (iCLK'event and iCLK = '1') then
127 r <= g;
128 end if;
129 end process P_SEQ;
130 end generate G_ASYNC;
131
132 end architecture TP;
さらに、何時もの様にやる気の無いテストベンチを作る。
1 library ieee;見ての通り、入力はLFSRとノコギリ波。
2 use ieee.std_logic_1164.all;
3 use ieee.std_logic_arith.all;
4 use work.MAF_PKG.all;
5
6 entity BENCH_MAF is
7 begin
8 end entity BENCH_MAF;
9
10 architecture BENCH of BENCH_MAF is
11
12 constant cIS_SYNC : boolean := true;
13 constant cDW : integer range 2 to integer'high := 12;
14 constant clog2N : integer range 1 to integer'high := 5;
15 constant cN : integer range 2 to integer'high := 2**clog2N;
16
17 constant cCLK_CYCLE : time := 1.0 us;
18 constant cCLR_TIME : time := (cN+1)*cCLK_CYCLE;
19
20 signal sCLK : std_logic := '0';
21 signal sCLR : std_logic := '1';
22 signal sLFSR_D : std_logic_vector(cDW-1 downto 0) := (others => '0');
23 signal sTRIANGLE_D : std_logic_vector(cDW-1 downto 0) := (others => '0');
24 signal sMAF_LFSR_oQ : std_logic_vector(cDW-1 downto 0);
25 signal sMAF_TRIANGLE_oQ : std_logic_vector(cDW-1 downto 0);
26
27 function fLFSR (
28 iD : std_logic_vector;
29 iDW : integer range 2 to integer'high
30 ) return std_logic_vector is
31 begin
32 if (iDW = 12) then
33 return (iD(7) xor iD(4) xor iD(3) xor iD(0)) & iD(11 downto 1);
34 else
35 assert (iDW = 12)
36 report "fLFSR: Call with non-supported iDW!!1" -- :P
37 severity warning;
38 return iD;
39 end if;
40 end function fLFSR;
41
42 function fTRIANGLE (
43 iD : std_logic_vector;
44 iDW : integer range 2 to integer'high
45 ) return std_logic_vector is
46 begin
47 if (signed(iD) <= -2**(iDW-1)) then
48 return conv_std_logic_vector(2**(iDW-1)-2**6, iDW);
49 else
50 return signed(iD) - 2**6;
51 end if;
52 end function fTRIANGLE;
53
54 begin
55
56 P_sCLK : process
57 begin
58 sCLK <= '0'; wait for cCLK_CYCLE/2;
59 sCLK <= '1'; wait for cCLK_CYCLE/2;
60 end process P_sCLK;
61
62 P_sCLR : process
63 begin
64 sCLR <= '1'; wait for cCLR_TIME;
65 sCLR <= '0'; wait;
66 end process P_sCLR;
67
68 P_sD : process (sCLK)
69 begin
70 if (sCLK'event and sCLK = '1') then
71 if (sCLR = '1') then
72 sLFSR_D <= (others => '1');
73 sTRIANGLE_D <= (others => '0');
74 else
75 sLFSR_D <= fLFSR(sLFSR_D, cDW);
76 sTRIANGLE_D <= fTRIANGLE(sTRIANGLE_D, cDW);
77 end if;
78 end if;
79 end process P_sD;
80
81 U_MAF_LFSR : MAF
82 generic map (
83 IS_SYNC => cIS_SYNC,
84 DW => cDW,
85 log2N => clog2N
86 )
87 port map (
88 iCLK => sCLK,
89 iCLR => sCLR,
90 iD => sLFSR_D,
91 oQ => sMAF_LFSR_oQ
92 );
93
94 U_MAF_TRIANGLE : MAF
95 generic map (
96 IS_SYNC => cIS_SYNC,
97 DW => cDW,
98 log2N => clog2N
99 )
100 port map (
101 iCLK => sCLK,
102 iCLR => sCLR,
103 iD => sTRIANGLE_D,
104 oQ => sMAF_TRIANGLE_oQ
105 );
106
107 end architecture BENCH;
前者をMAF.vhdl、後者をBENCH_MAF.vhdlとして、GHDLとGTKWaveでモニョる。workディレクトリを作って、analyzeして、elaborate。
$ mkdir workGHWなwaveファイルを吐くオプションを付けて500[us]くらいrunさせてー、GTKWaveでモニョモニョ。
$ ghdl -a --std=02 --workdir=./work --ieee=synopsys MAF.vhdl
$ ghdl -a --std=02 --workdir=./work --ieee=synopsys BENCH_MAF.vhdl
$ ghdl -e --std=02 --workdir=./work --ieee=synopsys -o BENCH_MAF-BENCH BENCH_MAF BENCH
$ ./BENCH_MAF-BENCH --stop-time=500us --wave=BENCH_MAF-BENCH-500us.ghw
$ gtkwave BENCH_MAF-BENCH-500us.ghw
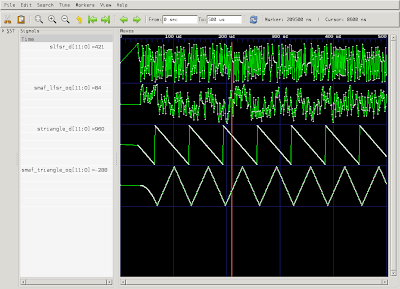
ハイ、いい感じに移動平均されているアルよ。 :)
以上の様に、結構いい感じのtwo-process手法だが、これ一本槍だと幾つか欠点があったりする。と言う感じで次回に続くのであったのであった。 :P